How continuous penetration testing reveals vulnerabilities that others miss through expert penetration testers, mature processes, a robust platform, and long-term partnership: continuous penetration testing reveals vulnerabilities that others miss.
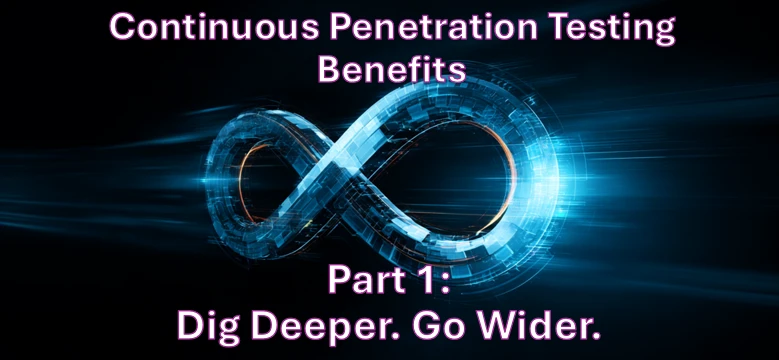
How continuous penetration testing reveals vulnerabilities that others miss through expert penetration testers, mature processes, a robust platform, and long-term partnership: continuous penetration testing reveals vulnerabilities that others miss.
A look into what the SOC process was designed for and how to determine if it is something you need.
For years, tabletop exercises (TTXs) have been a go-to method for testing an organization’s cybersecurity response plan. But as cyber threats evolve and real-world attacks grow more complex, so too...
Your organization has invested millions in cybersecurity tools. You've deployed next-generation firewalls, implemented endpoint protection, and built a Security...
A semi-technical look at how CASM, the platform powering Continuous Penetration Testing, uses machine learning to discover attack surface assets.
Legacy IT infrastructure represents one of the most significant yet understated risks to modern business operations. While these aging systems continue functioning day-to-day, they're silently...
In the rapidly changing world of cybersecurity, one truth remains: passwords continue to be a primary target for attackers. Even as new authentication methods emerge, many organizations still rely...
Why Your Pentest May Not Be Enough In today’s rapid change, cybercriminals continuously refine their tactics, techniques, and procedures (TTPs) to exploit vulnerabilities and evade detection. For...
From Zero to “Not Too Bad” in Two Months When I started working on Hash Master 1000, I had a vision of what I needed, but I lacked the capability to develop it myself. I wanted a single tool that...
Password cracking has come a long way, but what about password analysis? Back in the day, Pipal was our go-to tool for basic statistics and base-word identification. In 2017, two of SynerComm’s...